- From C# Reference for VS 2010
- example
- int? x = null;
- int y = x ?? -1;
- string s = null;
- Console.WriteLine (s ?? "Unspecified");
- used to define a default value for a nullable value type
- it returns the left-hand operand if it is not null; otherwise it returns the right operand
- more example from C# Programming Guide for VS 2010
- not in Java, but some would like to add the feature
- more about not in Java
- coalescing mean "to grow together"
- does this sound like a good description of what the operator is doing?
Regions in Java
I know the above picture is not about 'Regions in Java' code, but it looked nice.
- Basically, there are no region pre-compiler directives in Java
- There is support in some IDEs
- NetBeans
- // <editor-fold defaultstate="collapsed" desc="Your Fold Comment">
- ...
- // <editor-fold>
- Eclipse
- does it automatically for methods, imports, etc.
- Not declaration like in NetBeans
- Could you add one?
- Emacs?
Velocity Android 2.0 Internet Table 7" $100

- almost what I want
- missing the camera
- Multi touch capacitive screen
Viewsonic gTablet 10.1" Android 2.2: $270

- This is what I want except the price and Android version
Simple C# Program
- the main routine starts with a Capital 'M' in C#
- starts with a lower case 'm' in Java
Listeners and Events (Actions) in Java
- lesson on writing event listeners
- action listener
- public class Beeper ... implements ActionListener {
- adding an action listener to something
- button.addActionLisener ( new Beeper() )
- implement the method that does the action
- public void actionPerformed (ActionEvent e) {
- complete Beeper example
Java Color Map
- color map in java
- Copyright
- Carnegie Mellon
- Sun
- Mitsubishi
Java List Interface and ArrayList Class
- Interface List <E>
- Class ArrayList<E>
- Example
- List list = Collections.synchronizedList (new ArrayList(...));
Java Collection Interface and Collections Class
- Interface Collection<E>
- static void sort (List list)
- reverse (List list)
Java Queue Interface and PriorityQueue Class
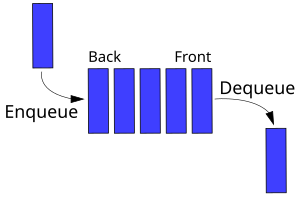
- new elements are put (enqueued) on the back of the queue
- element are pulled (dequeued) from the front of the queue
- First In First Out (FIFO)
- Interface Queue<E>
- offer (E o) // enqueue
- adds an element to the tail of the queue
- poll () // dequeue
- returns a removed element from the queue
- null is empty
- Class PriorityQueue<E>
- boolean add (E o)
- boolean offer (E o)
- E poll ()
Java Map Interface and HashMap Class
- Interface Map<K,V>
- V put (K key, V value)
- V get (Object key)
- Class HashMap<K,V>
- Example:
- Map m = Collections.synchronizedMap (new HashMap (...));
Chrome Remote Desktop
- BETA
- allow users to remotely access another computer through the Chrome browser or Chromebook
- An add on
- can easily miss the Icon after installation